Foods that boost your child’s brain
Fuel Code
Leaderboard1
Leaderboard2
Monday, July 16, 2018
Sunday, June 1, 2014
Hibernate One-To-One Mapping Tutorial
In this example you will learn how to map one-to-one relationship using Hibernate. Consider the following relationship between Student and Address entity.
Create these 2 files, Student and Address.
<?xml version="1.0"?>
<!DOCTYPE hibernate-mapping PUBLIC
"-//Hibernate/Hibernate Mapping DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd">
<hibernate-mapping>
<class name="com.akash.Student" table="STUDENT">
<meta attribute="class-description">This class contains student details.</meta>
<id name="studentId" type="long" column="STUDENT_ID">
<generator class="native" />
</id>
<property name="studentName" type="string" not-null="true" length="100" column="STUDENT_NAME" />
<many-to-one name="studentAddress" class="com.akash.Address" column="STUDENT_ADDRESS" not-null="true" cascade="all" unique="true" />
</class>
</hibernate-mapping>
Please post comments !
Share this:
According to the relationship each student should have a unique address.
To create this relationship you need to have a STUDENT and ADDRESS table. The relational model is shown below.
According to the relationship each student should have a unique address.
To create this relationship you need to have a STUDENT and ADDRESS table. The relational model is shown below.

Create these 2 files, Student and Address.
Student.java
public class Student implements java.io.Serializable {
private long studentId;
private String studentName;
private Address studentAddress;
public Student() {}
public Student(String studentName, Address studentAddress) {
this.studentName = studentName;
this.studentAddress = studentAddress;
}
public long getStudentId() {
return this.studentId;
}
public void setStudentId(long studentId) {
this.studentId = studentId;
}
public String getStudentName() {
return this.studentName;
}
public void setStudentName(String studentName) {
this.studentName = studentName;
}
public Address getStudentAddress() {
return this.studentAddress;
}
public void setStudentAddress(Address studentAddress) {
this.studentAddress = studentAddress;
}
}
private long studentId;
private String studentName;
private Address studentAddress;
public Student() {}
public Student(String studentName, Address studentAddress) {
this.studentName = studentName;
this.studentAddress = studentAddress;
}
public long getStudentId() {
return this.studentId;
}
public void setStudentId(long studentId) {
this.studentId = studentId;
}
public String getStudentName() {
return this.studentName;
}
public void setStudentName(String studentName) {
this.studentName = studentName;
}
public Address getStudentAddress() {
return this.studentAddress;
}
public void setStudentAddress(Address studentAddress) {
this.studentAddress = studentAddress;
}
}
Address.java
public class Address implements java.io.Serializable {
private long addressId;
private String street;
private String city;
private String state;
private String zipcode;
public Address() {}
public Address(String street, String city, String state, String zipcode) {
this.street = street;
this.city = city;
this.state = state;
this.zipcode = zipcode;
}
public long getAddressId() {
return this.addressId;
}
public void setAddressId(long addressId) {
this.addressId = addressId;
}
public String getStreet() {
return this.street;
}
public void setStreet(String street) {
this.street = street;
}
public String getCity() {
return this.city;
}
public void setCity(String city) {
this.city = city;
}
public String getState() {
return this.state;
}
public void setState(String state) {
this.state = state;
}
public String getZipcode() {
return this.zipcode;
}
public void setZipcode(String zipcode) {
this.zipcode = zipcode;
}
}
private long addressId;
private String street;
private String city;
private String state;
private String zipcode;
public Address() {}
public Address(String street, String city, String state, String zipcode) {
this.street = street;
this.city = city;
this.state = state;
this.zipcode = zipcode;
}
public long getAddressId() {
return this.addressId;
}
public void setAddressId(long addressId) {
this.addressId = addressId;
}
public String getStreet() {
return this.street;
}
public void setStreet(String street) {
this.street = street;
}
public String getCity() {
return this.city;
}
public void setCity(String city) {
this.city = city;
}
public String getState() {
return this.state;
}
public void setState(String state) {
this.state = state;
}
public String getZipcode() {
return this.zipcode;
}
public void setZipcode(String zipcode) {
this.zipcode = zipcode;
}
}
Generate Hibernate mapping files, using Hibernate/JBoss tool. To generate code using Hibernate Tools refer this example )
The following classes will be generated.
Student.hbm.xml
<!DOCTYPE hibernate-mapping PUBLIC
"-//Hibernate/Hibernate Mapping DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd">
<hibernate-mapping>
<class name="com.akash.Student" table="STUDENT">
<meta attribute="class-description">This class contains student details.</meta>
<id name="studentId" type="long" column="STUDENT_ID">
<generator class="native" />
</id>
<property name="studentName" type="string" not-null="true" length="100" column="STUDENT_NAME" />
<many-to-one name="studentAddress" class="com.akash.Address" column="STUDENT_ADDRESS" not-null="true" cascade="all" unique="true" />
</class>
</hibernate-mapping>
Address.hbm.xml
<?xml version="1.0"?>
<!DOCTYPE hibernate-mapping PUBLIC
"-//Hibernate/Hibernate Mapping DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd">
<hibernate-mapping>
<class name="com.akash.Address" table="ADDRESS">
<meta attribute="class-description">This class contains the student's address
details.</meta>
<id name="addressId" type="long" column="ADDRESS_ID">
<generator class="native" />
</id>
<property name="street" column="ADDRESS_STREET" type="string" length="250" />
<property name="city" column="ADDRESS_CITY" type="string" length="50" />
<property name="state" column="ADDRESS_STATE" type="string" length="50" />
<property name="zipcode" column="ADDRESS_ZIPCODE" type="string" length="10" />
</class>
</hibernate-mapping>
Hibernate configuration file:
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE hibernate-configuration PUBLIC
"-//Hibernate/Hibernate Configuration DTD 3.0//EN"
"http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<property name="hibernate.connection.driver_class"> org.hsqldb.jdbcDriver </property>
<property name="hibernate.connection.url"> jdbc:hsqldb:hsql://localhost</property>
<property name="hibernate.connection.username">sa</property>
<property name="connection.password"></property>
<property name="connection.pool_size">1</property>
<property name="hibernate.dialect"> org.hibernate.dialect.HSQLDialect </property>
<property name="show_sql">true</property>
<property name="hbm2ddl.auto">create-drop</property>
<mapping resource="com/akash/Student.hbm.xml"/>
<mapping resource="com/akash/Address.hbm.xml"/>
</session-factory>
</hibernate-configuration>
Create a main class to run this:
package com.akash;
import org.hibernate.HibernateException;
import org.hibernate.Session;
import org.hibernate.Transaction;
import com.vaannila.util.HibernateUtil;
public class Main {
public static void main(String[] args) {
Session session = HibernateUtil.getSessionFactory().openSession();
Transaction transaction = null;
try {
transaction = session.beginTransaction();
Address address1 = new Address("OMR Road", "Chennai", "TN", "600097");
Address address2 = new Address("Ring Road", "Banglore", "Karnataka", "560000");
Student student1 = new Student("Eswar", address1);
Student student2 = new Student("Joe", address2);
session.save(student1);
session.save(student2);
transaction.commit();
} catch (HibernateException e) {
transaction.rollback();
e.printStackTrace();
} finally {
session.close();
}
}
}
On executing the Main class you will see the following output.
The Student table has two records.

The Address table has two record.

Each student record points to a different address record, this illustrates the one-to-one mapping.
Please post comments !
Share this:
Hibernate Introduction
In this tutorial am going to explain, why Hibernate came into picture though we have JDBC for connecting to the database, and what is this hibernate frame work first let us see what are the draw backs of JDBC.
Draw Backs of JDBC:
1. In JDBC, if we open a database connection we need to write in try, and if any exceptions occurred catch block will takers about it, and finally used to close the connections.
2. Here as a programmer we must close the connection, or we may get a chance to get our of connections message…!
3. Actually if we didn’t close the connection in the finally block, then jdbc doesn’t responsible to close that connection.
4. In JDBC we need to write Sql commands in various places, after the program has created if the table structure is modified then the JDBC program doesn’t work, again we need to modify and compile and re-deploy required, which is tedious.
5. JDBC used to generate database related error codes if an exception will occurs, but java programmers are unknown about this error codes right.
6. In the Enterprise applications, the data flow with in an application from class to class will be in the form of objects, but while storing data finally in a database using JDBC then that object will be converted into text. Because JDBC doesn’t transfer objects directly.
What is Hibernate in Java? - Hibernate is ORM tool in Java.
Are you beginner in ORM framework in Java programming Language? Are you looking for the information about the Hibernate ORM? Then this is the best place to learn about Hibernate Framework in Java. The Hibernate in Java is framework used for developing the data access layer in Java based applications. This article gives you the complete information about Hibernate framework in Java.
There are many different databases such as Oracle, MySQL, MS SQL Server etc... Developers are using JDBC to develop the code for database interaction between application and the database. JDBC programming requires lot of work to create SQL statement, execute the statement and then process the results. It is the responsibility of the programmer to handle the exceptions occurred in runtime. Exception handling is also a very big task and it requires a lot of effort to write good code. To solve all these issues ORM tool was developed. The ORM tool stands for Object Relational Mapping tool and it takes care to generating the sql statements, executing the sql statement and finally processing the result. It also handles the exceptions occurred in the program. Hibernate is one of the ORM tool.
Features of ORM:
Here are the features and benefits of ORM tools:
1. The ORM tool is great tool which is used to map the logical business model (POJO class) with the relational database (physical model).
2. The ORM tool is very useful in reducing the amount of coding. ORM tools helps the developers to quickly develop the data access layer. Developers mostly concentrate on the business functions and uses the ORM tool for CRUD (Create, Read, Update and Delete) operation.
3. Easy to adapt the changes in database structure. If you have to change the database structure you just have to change the definition of model class the ORM will automatically change the SQL and Select queries. This eases the development and maintenance of enterprise application very easily.
4. The ORM tools provides rich query language which based on the domain model (POJO) class. Developers writes the query using the domain model and the the ORM tool generates the SQL queries automatically. So, programmer concentrates on the business login and the ORM tool takes care of the persistence, retrieval and query the database.
5. ORM tool support relationship amongst the objects.
6. It supports lazy loading and many other optimization functions.
7. It also supports the concurrency.
8. It also supports primary and secondary level cache.
9. Transaction management also a very important feature of the database. The ORM tools also supports transaction management and error handling.
Hibernate components
Hibernate framework includes following components which makes it pluggable and you can use the components you just need.
1. Hibernate Core: This is also know as Hibernate ORM. Hibernate Core is the main component of the Hibernate architecture. This components is responsible for performing the CRUD operations.
2. Hibernate Annotations: This is merged with Hibernate Core in the release of Hibernate version 3.6. Hibernate Annotation is a way to provide the mapping metadata to the Hibernate runtime.
3. Hibernate Entity Manager: The HibernateEntity Manager provides the API for performing the CRUD operations. Though HibernateEntity manager programmer interacts with the database.
4. Hibernate Envers: Is and Easy Entity Auditing library.
5. Hibernate OGM: The OGM stands for Object/Grid Mapper which is an extension to store data in a NoSQL store.
6. Hibernate Shards: The Hibernate Shards is used for horizontal partitioning for multiple relational databases. Although Hibernate Shards is not fully compatible with Hibernate 4.x. Check the compatibility in the current release specs.
7. Hibernate Search: The Hibernate Search is hibernate library to access the Apache Lucene full text engine from Hibernate.
8. Hibernate Tools: It includes the Eclipse plugins, Ant tasks etc...
9. Hibernate Validator: Used for validation in the POJO class.
How does hibernate code looks like?
Session session = getSessionFactory().openSession();
Transaction tx = session.beginTransaction();
MyPersistanceClass mpc = new MyPersistanceClass ("Sample App");
session.save(mpc);
tx.commit();
session.close();
What is a hibernate xml mapping document and how does it look like?
In order to make most of the things work in hibernate, usually the information is provided in an xml document. This document is called as xml mapping document. The document defines, among other things, how properties of the user defined persistence classes’ map to the columns of the relative tables in database.
<?xml version="1.0"?>
<!DOCTYPE hibernate-mapping PUBLIC
"http://hibernate.sourceforge.net/hibernate-mapping-2.0.dtd">
<hibernate-mapping>
<class name="sample.MyPersistanceClass" table="MyPersitaceTable">
<id name="id" column="MyPerId">
<generator class="increment"/>
</id>
<property name="text" column="Persistance_message"/>
<many-to-one name="nxtPer" cascade="all" column="NxtPerId"/>
</class>

Please post comments !
Share this..
Friday, May 30, 2014
How to use shutdown command under Ubuntu Linux
I this post i have listed out some commands to shutdown Ubuntu Linux using a commnd.
Open your terminal with CTRL+ALT+T and do these following commands
Shutdown command
shutdown arranges for the system to be brought down in a safe way. All logged-in users are notified that the system is going down and, within the last five minutes of TIME, new logins are prevented. The shutdown utility provides an automated shutdown procedure for supersers to nicely notify users when the system is shutting down, saving them from system administrators, hackers, and gurus, who would otherwise not bother with such niceties.
How do I use shutdown command?
The shutdown command can be used to turn off or reboot a computer. Type the command as follows to shutdown server / computer immediately:
$ sudo shutdown -h now
OR$ sudo shutdown -h 0
How do I shutdown compute at specific time?
To shutdown computer at 6:45pm, enter:
$ sudo shutdown -h 18:45 "Server is going down for maintenance"
At 6:30pm message will go out to all user and 6:45 system will shutdown.
Please note that you can also use halt or poweroff or reboot command for stopping and restarting the system:
$ sudo halt
OR$ sudo poweroff
How do I reboot computer?
Simply use reboot command:
$ sudo reboot
OR$ sudo shutdown -r 0
Post Comments !
Share this article..
How To Generate Hibernate Mapping Files & Annotation With Hibernate Tools In Eclipse (Reverse Engineering)
In this article, we show you how to use Hibernate / JBoss Tools to generate Hibernate mapping files (hbm) and annotation code from database automatically.
Note: As i have used Maven to build project structure, so Maven already created 3 package for me:
2. New Hibernate Configuration
Select your project. Click on new for creating database connection, You need mysql-coonector-java-5.1.30.jar for this, so download it from mysql website.
Test Connection.. and click finish.
Now creating configurtion file, click on setup
Click on Apply and OK..
3. Hibernate Code Generation
Now, you are ready to generate the Hibernate mapping files and annotation codes.
- In “Hibernate Perspective”, click "Run As.." - > “Hibernate code generation” icon (see below figure) and select “Hibernate Code Generation Configuration”.
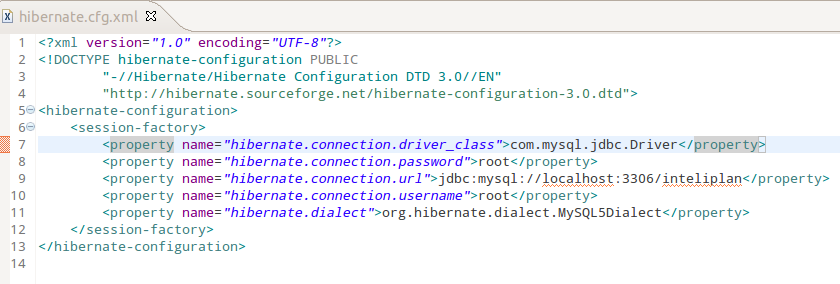
- Create a new configuration, select your “console configuration” (configured in step 2), puts your “Output directory” and checked option “Reverse engineer from JDBC Connection“.
- In “Exporter” tab, select what you want to generate, Model , mapping file (hbm) , DAO, annotation code and etc.
In my database 3 tables were present, here 3 hibernate mapping have been created. So finally we have created Hibernate Mapping files by using Eclipse reverse engineering.
Please post comments !
Tools required:
Eclipse v3.6 (Helios)
JBoss / Hibernate Tools v3.2
Oracle 11g
JDK 1.6
1. Open Hibernate Perspective
Open your “Hibernate Perspective“. In Eclipse IDE, select “Windows” >> “Open Perspective” >> “Others…” , choose “Hibernate“.
Note: As i have used Maven to build project structure, so Maven already created 3 package for me:
src/test/java
src/main/java
src/main/resources
If you haven't used Maven, then you can create these folders manually.
"src/main/resources" folder is required mostly for keeping hibernate file or other files which help in project configuration.
2. New Hibernate Configuration
In Hibernate Perspective, right click and select “Add Configuration”
In “Edit Configuration” dialog box,
In “Project” box, click on the “Browse..” button to select your project.
In “Database Connection” box, click “New..” button to create your database settings.
In “Configuration File” box, click “Setup” button to create a new or use existing “Hibernate configuration file”, hibernate.cfg.xml.
Select your project. Click on new for creating database connection, You need mysql-coonector-java-5.1.30.jar for this, so download it from mysql website.
Test Connection.. and click finish.
Now creating configurtion file, click on setup
Click on Apply and OK..
3. Hibernate Code Generation
Now, you are ready to generate the Hibernate mapping files and annotation codes.
- In “Hibernate Perspective”, click "Run As.." - > “Hibernate code generation” icon (see below figure) and select “Hibernate Code Generation Configuration”.
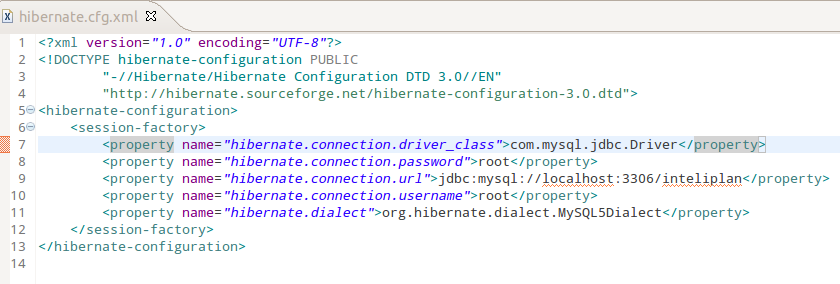
- Create a new configuration, select your “console configuration” (configured in step 2), puts your “Output directory” and checked option “Reverse engineer from JDBC Connection“.
- In “Exporter” tab, select what you want to generate, Model , mapping file (hbm) , DAO, annotation code and etc.
In my database 3 tables were present, here 3 hibernate mapping have been created. So finally we have created Hibernate Mapping files by using Eclipse reverse engineering.
Please post comments !

Subscribe to:
Posts (Atom)